Constructor in java is used in creation and initialization of object of a class inside memory. It's the constructor that provides the initial values of instance variables for an object inside memory. Constructors declarations are similar as method declarations except that constructors don't have any return type and their name is same as class name. The syntax of declaring a constructor is :
class
MyClassName {// No-argument constructor
Access_Modifier MyClassName() {// Initialization code
}// Parameterized constructor
Access_Modifier MyClassName(DataType param1, DataType param2 ...) {// Initialization code
} } Example :class
Person { Person() {// Initialization code
}public
Person(int
param1, String param2) {// Initialization code
} }
Access modifier of a constructor can be public, private, protected
or default(no modifier). These modifiers with constructors decides the
accessibility(visibility) of the constructor within or outside the class. After access modifier it's the name of constructor which must be same as the class name.
Parameters in constructors are optional, a constructor may or may not have parameters. A constructor without parameter is also known as no-argument constructor while a constructor with parameter is also known as parameterized constructor. The data type of parameters can be primitive or non-primitive.
In constructor body generally we initialize instance variables with some values but it's not limited to that only. You can use other statements as well as you do in methods.
You can define multiple constructors with different argument lists. Java differentiates constructors on the basis of the number of arguments in the list and their types. This is also known as constructor overloading. You cannot write two constructors that have the same number and type of arguments for the same class, because java compiler would not be able to differentiate them. In this case compiler will throw error.
Types of Constructors in Java
Constructor can be divided in three types.
- No-argument constructor
- Parameterized constructor
- Default constructor
We have already covered the definition of first two constructor. We will see how to use them in program example given below. We will cover the default constructor later in this tutorial.
How constructors are invoked(called) ?
Constructors are invoked automatically when the code that creates object of a class using new
keyword is executed.
Constructor Program in Java
class
Person {int
age;int
height; String name;// No-argument constructor
public
Person() { System.out.println("Initialization using no-argument constructor"
); age = 15; height = 160; name ="Pradeep"
; }// Parameterized constructor
public
Person(int
a,int
h, String n) { System.out.println("Initialization using parameterized constructor"
); age = a; height = h; name = n; }public static void
main(String [] args) { Person p1 =new
Person();// invokes no-argument constructor
p1.print(); Person p2 =new
Person(20,170,"Rahul"
);// invokes parameterized constructor
p2.print(); }void
print() { System.out.println("age = "
+age+", height = "
+height+", name = "
+name); } }
Output:
Initialization using no-argument constructor
age = 15, height = 160, name = Pradeep
Initialization using parameterized constructor
age = 20, height = 170, name = Rahul
How constructor works in Java
Let's understand this by above program. Once the code
is executed, it creates the object of new
Person();Person
class
inside memory and invokes the no-argument constructor which executes the given print statement and assigns values of instance
variables for this object. Finally the reference of this object is assigned into p1. Similarly code
creates another object inside memory and invokes the matching parameterized constructor which assigns
values passed to it in corresponding instance variables for object p2. So both objects will have their specific values of instance variables. You can imagine the object p2 inside memory
like below.new
Person(20,170,"Rahul"
);
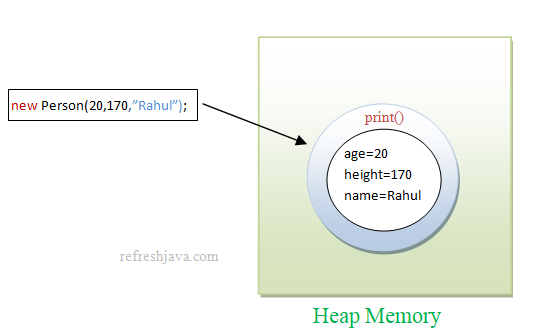
Can I create a class that have only no-argument or parameterized constructors ?
Yes you can create a class that have only no-argument constructor or only parameterized constructor. While creating the object you just have to ensure that the class contains the
matching constructor otherwise your program won't compile. For example if you try to create an object of Person
class in above program like below,
compiler will throw error since it would not find the matching constructor.
// Both statements will result in compilation error as it won't find matching constructor
Person p3 =
new
Person(20,"Rahul"
);Person p4 =
new
Person(20, 170.5,"Rahul"
);
What is default constructor
If you don't write any constructor in your class, java compiler inserts a no-argument constructor in your .class
file after compilation of your program. This constructor is known
as default constructor since it is provided by java compiler. For a class Person
having no constructors defined, the default constructor will look like below.
Person() { }
The default constructor assigns default values for non-initialized instance variables of an object, for example an integer instance variable will have value as 0 while a string variable will have value as null etc.
If you have created any constructor(no-argument or parameterized) in your class, java won't provide default constructor to your class. Constructors created by programmers is also known as user defined constructor.
Is no-argument constructor is same as default constructor ?
Default constructor is also a constructor without argument but if you have created a no-argument constructor in your class that will be a user defined constructor, not default constructor. Sometimes programmers get's confused about the same.
What is implicit and explicit constructor ?
Default constructor is also known as implicit constructor while constructors defined by programmer's in a class is known as explicit constructor.
Can we invoke constructors like methods ?
Constructors can not be invoked like methods. Constructors are invoked automatically. Though you can call one constructor from other constructor of same class using
this
keyword like below.
public
Person() {this
(15,160,"Pradeep"
);// invokes parameterized constructor
}public
Person(int
a,int
h, String n) { age = a; height = h; name = n; }
Similarly you can call other constructors if you have in your class by passing the number of arguments. To call no-argument constructor use this
().
If you are calling a constructor using this
keyword, ensure that it's the first statement in constructor body otherwise compiler will throw error.
We will get to know more about this
keyword in later tutorials.
What is private constructor ?
A constructor declared with private
modifier is known as private constructor. If a class contains only private constructor, you can not create object of that
class outside the class since your constructor won't be accessible(visible) outside the class.
Difference between Constructor and Method
Constructor | Method |
---|---|
Constructor name must be same as the class name. | Method name may or may not be same as class name. |
Constructor must not have return type. | Method must have return type. |
Constructor is used to initialize the state of an object. | Method is used to expose behavior of an object. |
Constructor is invoked(called) implicitly. | Method is invoked explicitly. |
Java compiler provides a default constructor if you don't declare any constructor in your class. | Method is not provided by java compiler in any case. |
You can not use keywords like abstract, final, static etc with constructors. |
These keywords can be used with methods. |
- A method can also have same name as class name but it must have return type which differentiates it from constructor.
- Declaring a method name same as class name is not a good programming style.
- Every class has a constructor whether it's a normal class or a abstract class.
- You can call a method inside a constructor as well.