It is a prerequisite for a programmer to first know the syntax of writing program in a particular programming language. Sometimes it get's confusing for beginners to understand what exactly the syntax is. So let's see the details about syntax in an easy way in this tutorial.
Every programming language defines a set of rules for writing programs in that programming language. These rules are known as syntax. Java has also defined a set of rules for defining different types of members(class, variable, methods etc) in a program, we call these rules as syntax in java. A java programmer should know these syntaxes before writing program in java.
Our real life languages has also defined a set of rules in order to write sentences in that language. For example English language has also defined a set of rules, which we call as Grammar. You should know the grammar first in order to write sentences in english, the same applies with programming languages as well.
In java a program generally contains a class. A class contains variables, methods,
constructors etc inside it. The program may also contain looping statements, array,
objects, if else
statements etc. Each of these members has some syntax which must be followed
while writing them inside a program. Let's see the basic syntax of some of
these members one by one. We will see the complete detail about these members in their respective tutorials.
Syntax of class in Java
In order to create a class in java, we should follow below syntax :
class
ClassName {// Define members of class like variables, method, constructors etc.
} Example:class
MyFirstJavaProgram {// Define members of class.
}// Below class declaration is incorrect, the class keyword must be in small letter.
Class
Test {// Define members of class.
}
Here class
is a keyword, used to define a class in java. ClassName
is the name of class, given by the programmer.
After the class name, it's the class body given inside { }. You can define variables, methods, constructors etc of this class inside the { }.
So in order to create a class in java you have to follow the above syntax. For more detail about class like what class is, how to create class in java, refer classes in java tutorial.
Is class keyword case-sensitive ?
All keywords in java are case-sensitive and they must be in small letter. So you can not use class
keyword as Class, CLASS, clAss etc, it must be in small letters.
Refer this tutorial to see the list of all keywords in java.
Syntax of variable in Java
In java if you have to create a variable, you have to follow below syntax :
DataType
variable_name = value; Example:int
age = 20;// Below variable declaration are incorrect
// variable name should not start with digits.
String
12message ="RefreshJava"
;// Variable name should come after data type
yearint
= 2021;
Each variable in java must have a data type. The data type of a variable basically tells what type of data that variable can store.
For example int
type of variable can store only integer type value, similarly
float
type of variable can store float type of values only. For more detail about data type, refer data type in java
tutorial.
The name of variable is given by the programmer which must be a valid identifier. There are some rules and conventions defined by java for naming your variable, refer identifiers naming tutorial to get detail about that. To get a complete detail about variables, refer variables in java tutorial.
Syntax of method in Java
In java if you want to create a method, you have to follow below syntax :
Return_Type methodName(DataType param1, DataType param2 ...) {// method logic or code.
return
some_value; } Example:int
add(int
num1,int
num2) {int
sum = num1 + num2;return
sum; }// Below method declaration is incorrect, () must be there after method name.
void
test {// method logic or code.
}
A method must have a return type which basically tells what type of value this method returns. The Return_Type of a method can be
primitive or non-primitive data type. If a method doesn't return a value, it's
return type must be void
or in other way if the return type of a method is void
, it means that method doesn't return any value.
After the return type, it's the name of the method which is given by the programmer. After method name, it's the parameters given inside () which are basically variables. Parameters are optional which means a method may or may not have parameters. You can access these parameters within the method body only, not outside the method body.
The data type of parameters can also be primitive or non-primitive. After parameters it's method body given inside { }
.
Everything given inside { } after method name are the part of that method. To get a complete detail about method refer methods in java tutorial.
What is syntax validation in Java
Syntax validation is a process in which java compiler(javac) validates whether the members of a program are defined as per the syntax or not. If it's not as per the syntax, the java compiler will show a syntax error for that member while compiling the program. In java once a program is written, it is compiled first using java compiler before it can be executed.
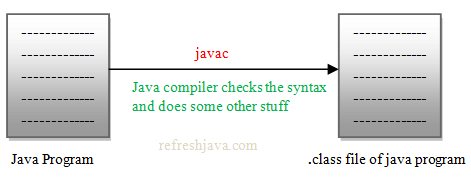
If there is any error in compilation, java programmer needs to fix that error first and then recompile the program. Only after successful compilation, the compiler generates a .class
file
of that program which is then used for execution of that program.
What is syntax error in Java ?
When a member of a program is not defined as per the syntax of that member, java compiler generates an error while compiling that program. We call this error as syntax error.
How do you find syntax errors ?
By compiling the program using java compiler we get the syntax errors of a program.
How do you fix a syntax error ?
Just follow the correct syntax of defining that member which is giving the syntax error.
Now you have learned some basic details about syntax in java, let's see how to write your first program in java in next tutorial which will help you to understand how to write program in java.