A package defined inside another package is known as sub package. Sub packages are nothing different than packages except that they are defined inside another packages. Sub packages are similar as sub directories which is a directory created inside another directory.
Sub packages in itself are packages, so you have to use/access them similar as packages. They can also have any types
like classes, interfaces, enums etc inside them.
How to create and use sub packages in Java
It's similar as creating packages in java. In previous tutorial, we created a package mypack
which is a directory in computer. Now if I create a
package(directory) testpack
inside mypack
directory, then package testpack
will be called as sub package.
Let's create a class inside this sub package. I have created a class MySubPackageProgram.java
as given below and saved it inside
D:\project\mypack\testpack
directory.
Subpackage program in Java
package
mypack.testpack;class
MySubPackageProgram {public static void
main(String args []) { System.out.println("My sub package program"
); } }
Notice here the package name is mypack.testpack
which is a subpackage. Let's compile and run this program by executing the commands given below.
If you don't know how to compile and execute package program, refer packages tutorial first.
javac mypack\testpack\MySubPackageProgram.java
java mypack.testpack.MySubPackageProgram
Output:
My sub package program
Why do we create Sub Packages
Subpackages are created to categorize/divide a package further. It's similar as creating sub folder inside a folder to categorize it further so that you can organize your content more better which will make easy to access the content. A package may have many sub packages inside it.
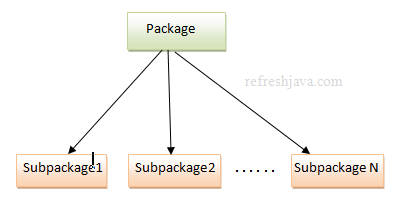
For an example in our computer generally we create directory like songs
to store songs inside it. Then inside that we may create sub directories like hindi songs
and
english songs
or old songs
and new songs
to categories the songs directory further. Doing this help us to organize or access
the songs easily. The same thing applies with sub packages as well.
How to import Sub packages
To access the classes or interfaces of a sub package, you need to import the sub package in your program first. Importing the parent package of a sub package doesn't import the sub packages classes or interfaces in your program. They must be imported explicitly in your program to access their classes and interfaces.
For example importing package mypack
in your program will not import the classes of sub package testpack
given above. Importing sub packages is same
as importing packages. The syntax of importing a sub package is :
// To import all classes of a sub package
import
packagename.subpackagename.*;// To import specific class of a sub package
import
packagename.subpackagename.classname; Exampleimport
mypack.testpack.*;import
mypack.testpack.MySubPackageProgram;
To get more detail about import statement, refer package import tutorial.
Example of Predefined SubPackages in Java
There are many predefined subpackages in java. Some of the examples of subpackages in java 8 are :
- The package
java
has subpackages likeawt, applet, io, lang, net, util
etc. The packagejava
doesn't have any class, interface, enums etc inside it. - The package
java.awt
has subpackages likecolor, font, image
etc inside it. The packagejava.awt
itself has many classes and interfaces declared inside it. - The package
java.util
has subpackages likeconcurrent, regex, stream
etc inside it. The packagejava.util
itself has many classes and interfaces declared inside it.
The program below shows how to use the Pattern
class of java.util.regex
subpackage. This sub package contains classes and interfaces for regular expressions.
The Pattern
class is generally used to search or match a particular pattern inside a given string.
import
java.util.regex.Pattern;// To imports all classes of java.util.regex subpackage.
// import java.util.regex.*;
class
PatternMatch {public static void
main(String args[]) {// Checks if the given string contains only alphabets
System.out.println(Pattern.matches("[a-zA-Z]*"
,"RefreshJava"
)); System.out.println(Pattern.matches("[a-zA-Z]*"
,"RefreshJava2"
));// Checks if the given string contains only numbers
System.out.println(Pattern.matches("[0-9]*"
,"123456"
)); System.out.println(Pattern.matches("[0-9]*"
,"123H456"
)); } }
Output:
true false true false
- Think of sub packages as packages while using or accessing them, they are nothing different.
- A sub package may have another sub packages inside it.
- Refer rt.jar file in bin folder of JRE to see some of the predefined sub packages in java.
- Though you can use capital letters as well for sub package name but prefer to use small letters only.
- If a package
mypack
contains a subpackage astespack
, then packagemypack
can not contain a class or interface with name astestpack
inside it.