Inheritance is one of the fundamental principle of object oriented programming. It is used quite often in java programming language. This tutorial covers different details about inheritance like what inheritance is, real world example of inheritance, how it is achieved in java, what are the advantages of using inheritance etc.
What is Inheritance
In general the meaning of inheritance is something that you got from your predecessor or parent, the same applies with java inheritance as well. Inheritance in java is a mechanism by which one class is allowed to inherit the features(fields and methods) of another class.
The class that inherits the feature of another class is known as subclass or child class and the class whose feature is being inherited is known as super class or parent class. By inheriting the parent class the child class get's the access of fields and methods of parent class.
More programmatically, inheritance feature allows the object of child class to acquire the properties and behavior of parent class, which simply means, using inheritance the object of child class get's the access of fields/methods of parent class even though those fields/methods are not defined in child class, they are defined only in parent class.
Real life example of inheritance
We can take parent and child relationship as an example of inheritance. The properties of parents like hands, legs, eyes, nose etc and the behaviors like walk, talk, eat, sleep etc are inherited in child, so child can also use/access these properties and behavior whenever needed.
Along with some common properties and behavior, both parent and child can have their specific or private properties as well, for example parent and child have their specific properties as blood group, date of birth etc and specific behavior like one plays cricket or any other game while other doesn't.
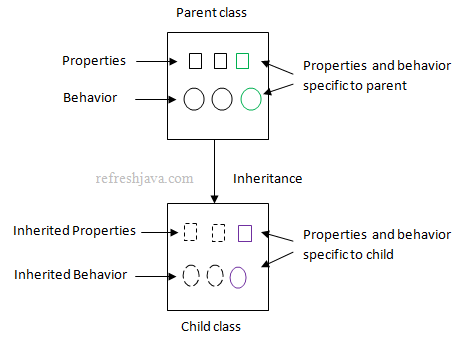
Generally in programming, the child classes will have more features than parent classes as they declare their own features as well apart from inheriting the parent classes features, so do not over indulge with real life example of inheritance.
How to implement inheritance in Java
Java provides extends
keyword to inherit the features of a class. Using extends
keyword your class can inherit the features of another class.
The basic syntax of inheriting a class in java is :
class
Aextends
B {// Here Class A will inherit the features of Class B
}
Here class A will inherit the features of class B which means object of class A will be able to access the variables and methods of class B. In addition to the access of superclass fields and methods, the subclasses can have their own fields and methods. Here class A is subclass or child class while class B is superclass or parent class.
Who decides whether to inherit a class or not ?
It's the programmer's who decides whether his class needs to inherit(extend) another class or not.
Important terminologies in Java inheritance
Subclass/Child class : The class that inherits the features of other class is known as subclass, also known as child class, derived class or extended class.
Superclass/Parent class : The class whose features are being inherited is known as super class, also known as parent class or base class.
Now let's see how we can inherit the features of a class using the program below :
Inheritance program in Java
class
B { String message ="This is class A variable"
;void
add(int
a,int
b) { System.out.println(a +"+"
+b+" = "
+(a+b)); } }class
Aextends
B {void
subtract(int
a,int
b) { System.out.println(a +"-"
+b+" = "
+(a-b)); }public static void
main(String args []) { A obj =new
A(); obj.add(5,10); System.out.println(obj.message); obj.subtract(20,10); } }
Output:
5+10 = 15 This is class A variable 20-10 = 10
Here you can see that the object of child class A is able to access the add
method and message
variable of class B, because the methods and variables of class B is
inherited in child class A using extends
keyword.
Can I access every member of parent class in child class ?
No, the accessibility of parent class members inside child class is also decided by access modifier. The child class can access only those variables/features of parent class
which has an access modifier that allows it to be accessed outside the class. For example a private
variable or method in parent class won't be
accessible inside child class.
What is IS-A relationship
IS-A relationship in java represents Inheritance. When there is an extends
or implements
keyword in the class declaration, then that specific class is said to be following the IS-A relationship. In other way when you see Is-A
relationship between two entities, you can use inheritance. For example :
- A student is a person.
- A car is a vehicle.
- A dog is an animal.
- A chair is a furniture.
Which means a class student
can extends a class person
, since student is a person. Similarly a class Car
can extends a class Vehicle
, since car is a
vehicle. Let's see this by the example below :
Student and Person class inheritance program
class
Person { String name ="Rahul"
;int
age = 20;void
talk() { System.out.println("I can talk"
); } }class
Studentextends
Person {int
rollno = 30;void
study() { System.out.println("I am studying"
); }public static void
main(String args []) { Student obj =new
Student(); System.out.println("Name : "
+obj.name+", age : "
+obj.age+", rollno : "
+obj.rollno); obj.talk(); obj.study(); } }
Output:
Name : Rahul, age : 20, rollno : 30 I can talk I am studying
When do we use inheritance in java ?
Though it's quite clear that when you want to reuse the features which is already created in some class, you should use inheritance, but as a good practice generally you should see if there is
is-a
relationship in both the classes or not, if yes then you should use inheritance there.
In other way, when you want to create a more specific version of a given class, you should use inheritance. For example a car is a more specific version of a vehicle because a vehicle can be of other types as well, like bike, bus, auto etc. In this case the class car can extend class vehicle. Furthermore if you want to create more specific version of a car like suv car or sedan car then you can use the inheritance between suvcar and car class as well.
More programmatically, inheritance comes into consideration, when there are certain classes which have common properties and behavior in them. You can put these common properties and behavior in a separate class and made it as generic class, the specific version classes can extend this class and reuse those properties and behaviors.
What we can do in subclasses ?
The subclasses can do everything that a normal class do along with inheriting the variables and methods of parent class, just to list down some of the things :
- We can declare new variable in the subclass that are not in the superclass.
- We can declare a variable in the subclass with same name as the one in superclass. In this case the subclass variable hides the superclass variable, thus the subclass doesn't inherit the variable from its superclass.
- We can write a new instance or static method in the subclass that are not in the superclass.
- We can write a new instance method in the subclass that has the same signature as superclass method. In this case the subclass does not inherit the method from its superclass. It is also known as method overriding.
- We can write a new static method in the subclass that has the same signature as the superclass method. In this case the subclass static method will hide the super class static method but it won't override that method.
- The subclass constructor implicitly invokes the constructor of superclass. We can call it explicitly as well using the
super
keyword. If calling explicitly, then it must be the first statement in your subclass constructor.
Advantages of Inheritance
- The biggest advantage of inheritance is code reusability, since the fields and methods of parent class get's inherited in child class, the child class won't have to create it again. It can access those features from parent class, that is what the code reusability is.
- It also help's to reduce code duplicacy. If inheritance is not used, multiple classes may need to write similar functions/logic in their body.
- Another advantages of inheritance is extensibility, you can add new features or change the existing features easily in subclasses.
- Using inheritance we can achieve runtime polymorphism(method overriding).
- Inheritance makes easy to maintain the code, as the common codes are written at one place.
What if I don't want my class to be inherited by any other class ?
Declare your class using final
keyword, eg. final class
A { ... }. Final classes can not be inherited.
We will see details of final
keyword in later tutorial.
- Every class in java internally extends Object class, so Object class is the super class of every class.
- If a class extends another class, then it won't extends Object class, instead it's parent class will inherit the Object class.
- A class can inherit only one class, as multiple inheritance is not allowed in java.
- The classes involved in inheritance can be in same or different packages.
- Static classes can also be inherited.
- You can not extends a class which is not visible(as per access modifier) in your class.
- If a class implements an interface, that is also a form of inheritance.