static
is a keyword in java which is used very often in java programs. Sometimes beginners faces difficulty to understand what exactly the static
keyword does and why to use this. This tutorial explains different usage of static
keyword and also covers different questions like when, how and why
do we use static
keyword in java.
Generally you need an object of a class to access members like variables and methods of that class, because these members belongs to object or instance of that class. Each object have their
specific values for such members. But sometimes we need members that should belong to class rather than objects and should be same for all the objects. To achieve this java provides
static
keyword. static
members belongs to class not objects. You can access such members using class name itself rather than objects.
Let's see the different usage of static
keyword.
static
variablestatic
methodstatic
blockstatic
inner class
In this tutorial we will discuss about static
variable and static
method only. For static block refer
initializer blocks tutorial and for static inner classes refer inner classes in java tutorial.
Apart from this there is static import in java which also uses static
keyword, refer package import tutorial for
static
import in java.
Java static variable
A static
variable is similar as normal variable except that it has a static
keyword in it's declaration.
static
variables are also called as class variables
as these variables belongs to class not objects. These variables must be created inside
class but outside any method or block. If you create static
variable inside any static or non-static
method or block, compiler will throw compilation error. The basic syntax of declaring a static
variable is :
class
className { access_modifierstatic
dataType varName; access_modifierstatic
dataType varName = value; } Example :class
Student {static
int
total;public static
String collegeName ="SUMVS"
; }
The access modifier of static
variable can be public, protected, private
or default(no modifier). The access modifier
decides visibility/accessibility of the variable within or outside the class. The data type of static
variable can be primitive or non-primitive data type.
The name of variable is given by the programmer. The value of
static
variable can be given while declaration or later in program. If static
variables are not initialized with any value,
java will assign a default value as per their data type.
Static variable memory allocation
As soon as java virtual machine loads your class inside memory,
static
variables of your class
are created inside the heap memory and it's created only once, it's not created separately for each object unlike instance variables.
As soon as the execution of your program finishes, static
variables are removed from memory.
static
variables belongs to class and is common to all objects of a class. There is only one copy of static
variable available
that is shared with all the objects of that class, if changes are made to that variable value using one object, all other objects will also see the effect of changes.
The image below shows how two objects obj1
and obj2
of Student
class shares a
static
variable collegeName
which is created only once.
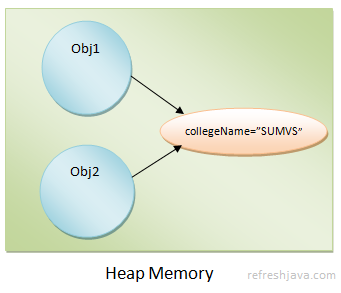
How to access static variable in Java
Since static
variables belongs to class, it can be accessed using class name as className.staticVarName
.
You can also access static
variable using object of a class as objName.staticVarName
but you should prefer to access
static
variable using class name as static
variables belongs to class not objects. You can access static
variables inside static or non-static methods or blocks.
You can access static
variables directly inside your class but outside the class(in other classes) you must need class name or object name to access the
static
variables of a class.
Static variable program in Java
class
Student {static
int
total = 40;static
String collegeName ="SUMVS"
; }class
StaticVarDemo {static
String test ="Testing static variable"
;public static void
main(String [] args) { System.out.println(test+", "
+ StaticVarDemo.test);// Accessing Student class static variables
System.out.println("Total students =
"+ Student.total); System.out.println("college name =
"+ Student.collegeName); } }
Output:
Testing static variable, Testing static variable
Total students = 40
college name = SUMVS
When to create static variable ?
You should create a static
variable when you need a variable that should be same for all the objects of a class or in other way you need a variable that is
not different for each objects. For example a Student
class can have a static
variable as collegeName
,
since it will be same for all the students of a college while variables like name, age, rollNo
etc can be declared as instance variables since
these will be different for each students.
Static method in Java
static
method is similar as normal method except that it has a static
keyword in it's declaration.
The basic syntax of declaring a static
method is :
access_modifierstatic
return_type methodName(DataType param1, DataType param2 ...) {// one or more line of code
return
some_value;// Needed if return_type is not void
} Example :public static
int
getArea() {// one or more line of code
return
some_value; }static
void
calculateVolume(double
radius) {// one or more line of code
}
The access modifier of a method can be public, protected, private
or default(no modifier). The access modifier of a method decides visibility/accessibility of a method
within or outside the class. The return type
of a method can be
primitive or non-primitive data type. If the method doesn't return a value, it's
return type must be void
. The name
of method is given by the programmer. Parameters are optional, a static
method may or may not have parameters.
How to access static method in Java
Since static
method belongs to class, you can access them using class name as className.methodName()
. You can also access
static
method using object as objName.methodName()
but you should prefer to access using class name as static
method belongs to class not objects. Static method can not access non-static variables or methods directly inside it's body, doing so will result in compilation error. You can create and access
local variables inside static method as you do in normal methods.
You can access static
methods directly inside your class but outside the class(in other classes) you must need class name or object name to access the
static
methods of a class.
Static method program in Java
class
Student {static int
total = 50;static
String collegeName ="SUMVS"
;public static void
printDetail() { System.out.println("total students = "
+ total); System.out.println("college name =
"+ collegeName); } }class
StaticMethodDemo {int
count = 20;static
String test ="Learning static method"
;public static void
main(String [] args) { StaticMethodDemo.testMethod();// testMethod(); // Can access testMethod directly as well.
Student.printDetail();// Calling printDetail method of Student class
// printDetail()
// Can't access other class static method directly.
}static void
testMethod() {// count = count+2;
// Static method can't access non-static variables
int
num = 30; System.out.println(test+", num = "
+num); } }
Output:
Learning static method, num = 30
total students = 50
college name = SUMVS
When to use static methods in Java
There are not any defined rules but there are some observations that can be used to create static
methods.
- You want to call method without creating instance of that class.
- If the method doesn't need to access/modify instance variables.
- If the method works only on arguments provided to it. For eg.
, this method only operates onstatic
int
factorial(int
number)number
provided to it. - If it's a kind of utility method. For eg. built-in methods of
Math
class likelog10(
etc. These are utilities that performs common operations, it doesn't require any object states.double
a), sqrt(double
a), cbrt(double
a)