This tutorial explains different types of variables in java, differences between them and their allocations in memory. The tutorial will help the beginners to use the correct variable type for their need in programs. In java there are three types of variables :
- Local variable
- Instance variable
- Static or Class variable
Java Local variable
Variables defined inside methods, constructors or blocks are called local variables. These variables are created in memory(stack memory) when execution of that method, constructor or block starts in which they are declared. These variables are accessible only within the method, constructor or block in which they are created. Local variables must be initialized with some value before their use otherwise compiler throws an error.
How to declare and access local variable in java
The declaration of local variable is same as normal variable, the only thing is that they are declared in method, constructor or block. In other way if you have declared variables in method, constructor or block they are basically the local variables in java. To access a local variable, you just have to use it's name. The program below shows how to create and access local variables in java.
Local variable program in java
class
LocalVariable {public
LocalVariable() {int
a = 10;// local variable a, created inside constructor
System.out.println("a = "
+a); } {int
b = 30;// local variable b, created inside block
System.out.println("b = "
+b); }public static void
main(String [] args) {int
c = 20;// local variable c, created inside method
System.out.println("c = "
+c);// Constructor and block will be executed by below line
LocalVariable lv =new
LocalVariable();int
d;// local variable d, created inside method
// Below code will throw error if uncommented, as d is not initialized.
// System.out.println(
} }"d = "
+d);
Output:
c = 20
b = 30
a = 10
When memory is allocated for local variables in Java ?
Memory for local variables are allocated as soon as the method, constructor or block containing the variable is executed.
What is a local variable default value in Java ?
Local variables don't have any default values in java, they must be initialized before they can be used.
Java Instance variable
Variables defined inside a class but outside any method, constructor or block are known as instance variable. Initialization of instance variable is not compulsory, if not initialized, these variables will have default values as per their data type
Instance variables, as the name suggests belongs to the instance or object of the class. Instance variables are created in memory(heap memory) as soon as the object of the class containing the instance variable is created. Every object of a class has it's own copy of instance variables, changes made to the variable value of one object doesn't reflect in other object.
How to declare and access instance variable in java
The declaration of instance variable is same as normal variable, the only thing is that they are declared inside class directly. In other way if you have declared variables inside a class directly, they are basically the instance variables in java
The Instance variables are accessed using object name like objName.instanceVarName
. You can also access the instance variable directly as well,
in this case java internally applies the object name for you. The good practice is to always use the object name for accessing the instance variable. The program below
shows how to declare and access instance variables in java.
Instance variable program in java
class
InstanceVariable {int
age = 20;// instance variable age, created inside class
String name ="Rahul"
;// instance variable name, created inside class
public static void
main(String [] args) { InstanceVariable iv =new
InstanceVariable(); System.out.println("Age = "
+iv.age); System.out.println("Name = "
+iv.name); } }
Output:
Age = 20
Name = Rahul
Class variable in Java
Variables defined inside a class(not inside method, constructor or block) using
static
keyword are known as static or class variables. Initialization of class
variable is also not compulsory, if not initialized, these
variables will have default values as per their
data type. These variables are known as class variables because they belong to class, not objects.
Class variables as the name suggests, belongs to class and is common to all objects of that class. These variables are created in memory as soon as the class containing the variable is loaded inside memory. You can refer static keyword tutorial to get complete detail about static or class variable in java.
How to declare and access class variable in java
The declaration of static or class variable is same as instance variable, except that they are declared along with static
keyword.
Static variable can be accessed using class name as className.staticVarName
or
using any of the object instances as well like objName.staticVarName
. The program below shows how to declare and access class variable in java.
Class variable program in java
class
ClassVariable {static
String classVar ="Class variable Test"
;// class variable classVar
public static void
main(String [ ] args) { System.out.println(ClassVariable.classVar); } }
Output:
Class variable Test
Difference between local, instance and static variable in java
Features | Local variable | Instance variable | Static variable |
---|---|---|---|
Declaration point | Inside a method, constructor, or block. | Inside the class. | Inside the class using static
keyword.
|
Lifetime | Created when method, constructor or block is
entered or executed. Destroyed on exit of method, constructor or block. |
Created when instance of class is created with new
keyword. Destroyed when object is available for garbage collection. |
Created when the program starts. Destroyed when the program execution completed. |
Scope/Visibility | Visible only in the method, constructor or block in which they are declared. | Visible to all methods in the class. It could be visible to other classes as well according to it's access modifier. | Same as instance variable |
Initial value | None. Must be assigned a value before it's use. | Default value as per Data Type.
0 for integers, false
for booleans, or null for object references etc. |
Same as instance variable. |
Use of Access Modifier | Access modifier(public , private ,
protected ) can not be
used with local variables |
Can be used. | Can be used |
Access from outside | Not possible, Local variable can be accessed within the method, constructor or block in which they are declared. | Instance variables can be accessed outside depending upon it's access modifier. It can be accessed using object of that class. | Same as instance variable. It can be accessed using object or class name. |
Memory Area | Stack memory. | Heap memory | Heap memory |
Use | Used for local computations | Used when value of variable must be referenced by more than one method | Generally used for declaring constants |
Java Program of local, instance and static variable
class
Student {static String
college = "SUMVS";// static or class variable college
int
rollNo;// instance variable rollNo
String
name;// instance variable name
public static void
main(String [] args) { Student s1 =new
Student();// local variable s1
s1.rollNo = 1; s1.name ="Ram"
; Student s2 =new
Student();// local variable s2
s2.rollNo = 2; s2.name ="Shyam"
; s1.printDetail(); s2.printDetail();int
count = 2;// local variable count
System.out.println("Total students =
"+ count); }void
printDetail() {int
count2 = 2;// local variable count2
System.out.println(rollNo +", "
+ name+", "
+ Student.college+", "+ count2); } }
Output:
1, Ram, SUMVS, 2
2, Shyam, SUMVS, 2
Total students = 2
Memory allocation for local, instance and static variables in Java
The image below shows where the memory is allocated for different types of java variables used in above program. The variable
count
and count2
are local variables, rollNo
and Name
are instance variables whereas college
is a class variable.
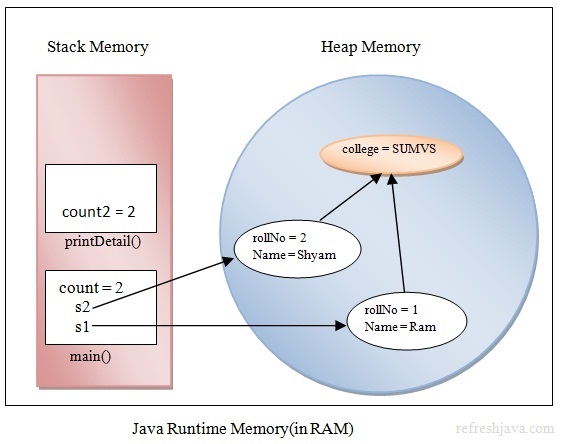
Can a local variable have the same name as an instance variable or static variable ?
Yes, you can declare a local variable with same name as an instance or static variable. When accessed within the local block, method or constructor, local variable will take precedence over instance or static variable.
- If a variable has to be used inside a single method only, it's good practice to create a local variable than instance or static variable.
- Compiler will throw error if you initialize a local variable conditionally (e.g. inside an if without else).
- Local variable is removed from stack memory as soon as the execution of method completed.
- Parameters in a method acts as local variable of that method.
- Instance variable is also called member or field variable.
- Declaring instance and static variable on top of the class is a good programming style.